Android Lifecycle - from the view of Compose
Learn more about not only the core stages of the activity lifecycle and how it impacts or changes with Jetpack Compose, but also how an activity reacts to hardware and software state changes!
Android lifecycle management has always been crucial for building reliable and performant apps, and it will only become more and more challenging as we add new software features like free-form windows and multiple split screens, as well as hardware features like foldable, rollable, and flip devices.
In this blog we will not only cover the core stages of the activity lifecycle and how it impacts or changes with jetpack compose but also try to cover how our activity reacts to hardware and software state changes.
Table Of Contents
- Activity Lifecycle
- Compose Lifecycle
- Lifecycle States
Activity Lifecycle
Android has multiple lifecycle states the android ComponentActivity()
provides many lifecycle states but 6 of the core states are onCreate()
, onStart()
, onResume()
, onPause()
, onStop()
, and onDestroy()
.
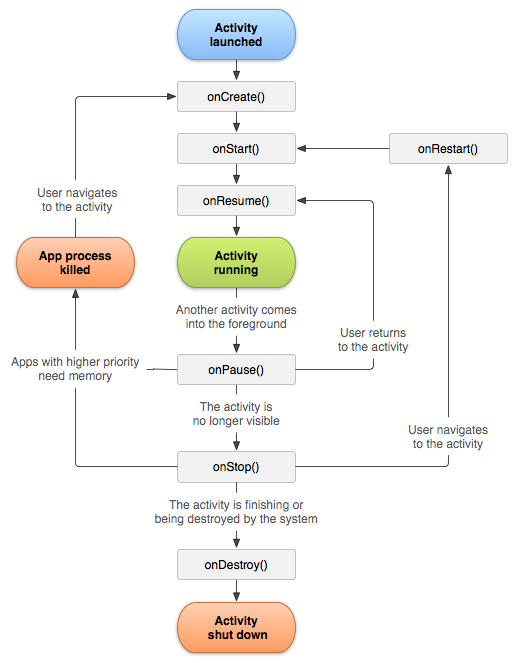
onCreate()
- It is fired when the system first creates the activity. A great time for startup logic that happens only once for the entire life of the activity.onStart()
- It is fired when the activity is visible to the user and the app prepares to enter the foreground and becomes interactiveonResume()
- It is fired when the app becomes interactive to the user and stays in this state until something happens to the activity or the screen gets turned offonPause()
- It is fired when the user leaves the activity or app, which doesn't necessarily mean the app is being destroyed. It indicates that the app is not currently in the foregroundonStop()
- It is fired when the app is no longer visible to the user or a newly created activity covers the entire screen.onDestroy()
- It is fired when the activity is destroyed. It can happen whenfinish()
is called or there is a configuration change like split-screen or rotation.
Lifecycle is important to make performant and efficient apps. If you want to get deeper into the topic check out official documentation.
Compose Lifecycle
Jetpack Compose does not deprecate or replace Activity Lifecycle. Compose is designed to be used as a single-activity architecture without fragments. To manage its own lifecycle, Compose introduces its own mechanisms, namely Recomposition and Side effects
- Recomposition - This happens when the UI is updated or the state of a composable change to reflect the change in the UI. As compose is a tree of nodes any state changes affect all the composables within the hierarchy.
- Side-effects - A side-effect is a change to the state of the app that happens outside the scope of composable function. It happens due to composable's lifecycle and properties such as recompositions executing in different orders, or recompositions that can be discarded. A composable should ideally be side-effect free. Jetpack compose provides different side-effect APIs like
LaunchedEffect
,DisposableEffect
,SideEffect
,ProduceState
,DerivedStateOf
.
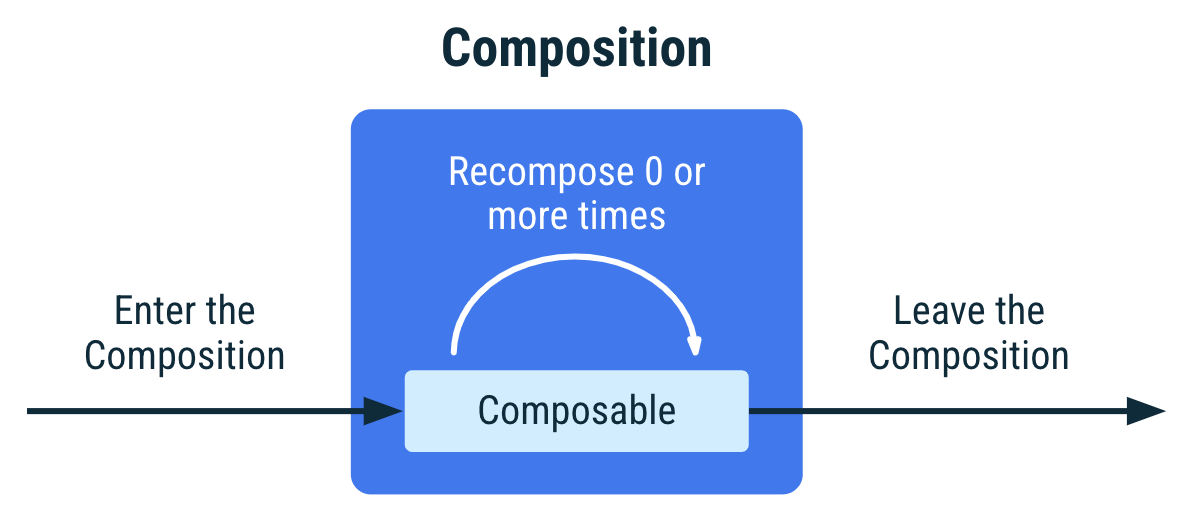
Recomposition and Side-effects aren't the only way to manage state in compose. Composable functions can use the remember
API to store object in memory. A value
computed is stored in cache memory. Any changes to value
schedules recomposition of any composable functions that read value
. Compose also supports the use of Flow
, LiveData
, and rxjava
.
But how do we keep track of the lifecycle in compose? we can use DisposableEffect
for it.
to learn more in detail check out this blog.
Lifecycle States
Below are a few real-world use cases that can happen to the lifecycle of an app XML or Compose.
Lifecycle states are listed in the order they are triggered.
- The App is Launched
- The app is Minized or the User Switches to a different app
- The Screen Rotation or Configuration Changes
- The app is in Split-screen mode
- The app is in Freeform
- The App is Killed
The App is Launched
ON_CREATE
ON_START
ON_RESUME
The app is Minized or the User Switches to a different app
ON_PAUSE
ON_STOP
Comes back to the app
ON_RESTART
ON_START
ON_RESUME
The Screen Rotation or Configuration Changes
ON_PAUSE
ON_STOP
ON_DESTROY
ON_CREATE
ON_START
ON_RESUME
The app is in Split-screen mode
ON_PAUSE
ON_STOP
ON_DESTROY
ON_CREATE
ON_START
ON_RESUME
The app is in Freeform
ON_PAUSE
ON_STOP
ON_DESTROY
ON_CREATE
ON_START
ON_RESUME
The App is Killed
ON_PAUSE
ON_STOP
ON_DESTROY
Conclusion
Understanding the lifecycle of the application is very crucial and helps you better manage app states and help mitigate a lot of development issues. A pro tip is to think prior about the lifecycle of the important components in the screen when working with compose (it saved a lot of time later). With that said hope you have a happy lifecycle ahead – and most importantly,
Happy Composing! :)
Bonus links
Publish your Android Library to Maven Central:
Animate your compose UI:
Learn about open-source licenses:
Learn about coroutines here:
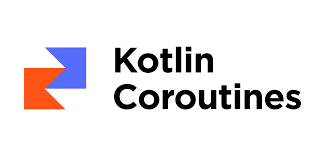